using django.
To create this application I have used django 1.5.0
First open a terminal or console.
So lets create a project called ‘myapp’ using the following command.
1
| django - admin.py startproject myapp |
So to do this first go to the myapp directory using ‘cd myapp’ then
enter the following command.
1
| django - admin.py startapp login |
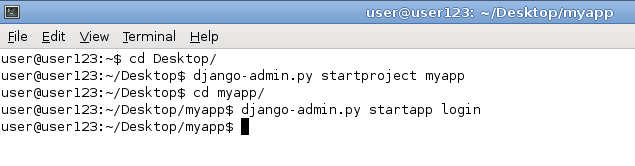
And this will create your application.
Next I have used a sqlite database for myapp. So now open the settings.py file (myapp > myapp > settings.py)
modify the following lines to use a database. And the name of the database file is loginapp.sqlite.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| import os BASE_DIR = os.path.dirname(os.path.abspath(__file__)) DATABASES = { 'default' : { 'ENGINE' : 'django.db.backends.sqlite3' , # Add 'postgresql_psycopg2', 'mysql', 'sqlite3' or 'oracle'. 'NAME' : BASE_DIR + '/loginapp.sqlite' , # Or path to database file if using sqlite3. # The following settings are not used with sqlite3: 'USER' : '', 'PASSWORD' : '', 'HOST' : ' ', # Empty for localhost through domain sockets or ' 127.0 . 0.1 ' for localhost through TCP. 'PORT' : '', # Set to empty string for default. } } |
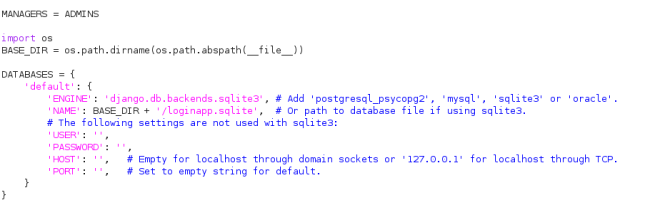
Now you need to sync the datadabe, to do this go to the terminal and enter the following command.And also
create a superuser.
1
| python manage.py syncdb |
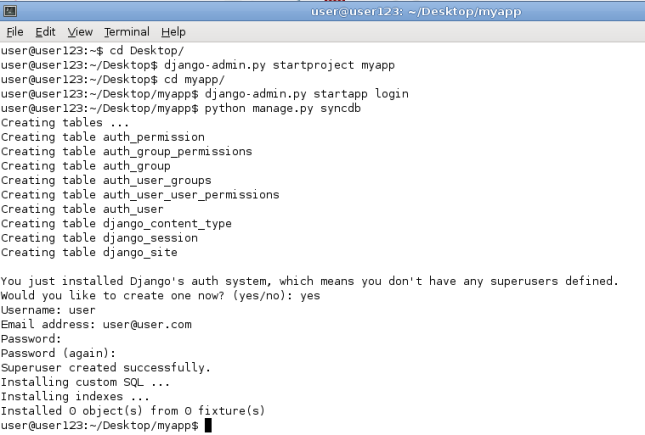
Now you need to define the path of your template directory in the settings.py file. To do this add the following line
into your TEMPLATE_DIRS directive.
1
2
3
4
5
6
| TEMPLATE_DIRS = ( # Put strings here, like "/home/html/django_templates" or "C:/www/django/templates". # Always use forward slashes, even on Windows. # Don't forget to use absolute paths, not relative paths. os.path.join(os.path.dirname(__file__), 'templates' ), ) |
1
2
3
4
5
6
7
8
9
10
11
12
13
| INSTALLED_APPS = ( 'django.contrib.auth' , 'django.contrib.contenttypes' , 'django.contrib.sessions' , 'django.contrib.sites' , 'django.contrib.messages' , 'django.contrib.staticfiles' , # Uncomment the next line to enable the admin: # 'django.contrib.admin', # Uncomment the next line to enable admin documentation: # 'django.contrib.admindocs', 'login' , ) |
a method that that requires login and the user is not logged in then it will redirect the user to login page.
1
2
| import django.contrib.auth django.contrib.auth.LOGIN_URL = '/' |
This code is used to create the registration form.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
| #files.py import re from django import forms from django.contrib.auth.models import User from django.utils.translation import ugettext_lazy as _ class RegistrationForm(forms.Form): username = forms.RegexField(regex = r '^\w+$' , widget = forms.TextInput(attrs = dict (required = True , max_length = 30 )), label = _( "Username" ), error_messages = { 'invalid' : _( "This value must contain only letters, numbers and underscores." ) }) email = forms.EmailField(widget = forms.TextInput(attrs = dict (required = True , max_length = 30 )), label = _( "Email address" )) password1 = forms.CharField(widget = forms.PasswordInput(attrs = dict (required = True , max_length = 30 , render_value = False )), label = _( "Password" )) password2 = forms.CharField(widget = forms.PasswordInput(attrs = dict (required = True , max_length = 30 , render_value = False )), label = _( "Password (again)" )) def clean_username( self ): try : user = User.objects.get(username__iexact = self .cleaned_data[ 'username' ]) except User.DoesNotExist: return self .cleaned_data[ 'username' ] raise forms.ValidationError(_( "The username already exists. Please try another one." )) def clean( self ): if 'password1' in self .cleaned_data and 'password2' in self .cleaned_data: if self .cleaned_data[ 'password1' ] ! = self .cleaned_data[ 'password2' ]: raise forms.ValidationError(_( "The two password fields did not match." )) return self .cleaned_data |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
| #views.py from login.forms import * from django.contrib.auth.decorators import login_required from django.contrib.auth import logout from django.views.decorators.csrf import csrf_protect from django.shortcuts import render_to_response from django.http import HttpResponseRedirect from django.template import RequestContext @csrf_protect def register(request): if request.method = = 'POST' : form = RegistrationForm(request.POST) if form.is_valid(): user = User.objects.create_user( username = form.cleaned_data[ 'username' ], password = form.cleaned_data[ 'password1' ], email = form.cleaned_data[ 'email' ] ) return HttpResponseRedirect( '/register/success/' ) else : form = RegistrationForm() variables = RequestContext(request, { 'form' : form }) return render_to_response( 'registration/register.html' , variables, ) def register_success(request): return render_to_response( 'registration/success.html' , ) def logout_page(request): logout(request) return HttpResponseRedirect( '/' ) @login_required def home(request): return render_to_response( 'home.html' , { 'user' : request.user } ) |
Next create a file called base.html inside templates folder and paste the following code in that file.
1
2
3
4
5
6
7
8
9
10
11
12
| <! DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> < html > < head > < title >Django Login Application | {% block title %}{% endblock %}</ title > </ head > < body > < h1 >{% block head %}{% endblock %}</ h1 > {% block content %}{% endblock %} </ body > </ html > |
1
2
3
4
5
6
7
| {% extends "base.html" %} {% block title %}Welcome to Django{% endblock %} {% block head %}Welcome to Django{% endblock %} {% block content %} < p >Welcome {{ user.username }} !!!</ p > < a href = "/logout/" >Logout</ a > {% endblock %} |
Create a file called login.html inside the registration folder and paste the following code in that file.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| {% extends "base.html" %} {% block title %}Login{% endblock %} {% block head %}Login{% endblock %} {% block content %} {% if form.errors %} < p >Your username and password didn't match. Please try again.</ p > {% endif %} < form method = "post" action = "." >{% csrf_token %} < table border = "0" > < tr >< th >< label for = "id_username" >Username:</ label ></ th >< td >{{ form.username }}</ td ></ tr > < tr >< th >< label for = "id_password" >Password:</ label ></ th >< td >{{ form.password }}</ td ></ tr > </ table > < input type = "submit" value = "Login" /> < input type = "hidden" name = "next" value = "/home" /> </ form > < a href = "/register" >Register</ a > {% endblock %} |
1
2
3
4
5
6
7
8
9
10
11
12
| {% extends "base.html" %} {% block title %}User Registration{% endblock %} {% block head %}User Registration{% endblock %} {% block content %} < form method = "post" action = "." >{% csrf_token %} < table border = "0" > {{ form.as_table }} </ table > < input type = "submit" value = "Register" /> </ form > < a href = "/" >Login</ a > {% endblock %} |
1
2
3
4
5
6
7
8
9
| {% extends "base.html" %} {% block title %}Registration Successful{% endblock %} {% block head %} Registration Completed Successfully {% endblock %} {% block content %} Thank you for registering. < a href = "/" >Login</ a > {% endblock %} |
1
2
3
4
5
6
7
8
9
10
11
| from django.conf.urls import patterns, include, url from login.views import * urlpatterns = patterns('', url(r '^$' , 'django.contrib.auth.views.login' ), url(r '^logout/$' , logout_page), url(r '^accounts/login/$' , 'django.contrib.auth.views.login' ), # If user is not login it will redirect to login page url(r '^register/$' , register), url(r '^register/success/$' , register_success), url(r '^home/$' , home), ) |
1
| python manage.py runserver |
http://127.0.0.1:8000/
No comments:
Post a Comment